In order to test the antimicrobial effect of the developed antimicrobial peptides, it is necessary to design biological experiments to evaluate the antimicrobial effects of antimicrobial peptides. Although we can inject Staphylococcus aureus into the intestines of experimental animals (such as mice, etc.), and inject drugs into mice, and observe the health status of mice, etc., the process is troublesome to do a large number of biological experiments, and due to some differences between the internal environment of the human intestine and the intestinal tract of mice, the experimental results obtained by using mice cannot well simulate the therapeutic effect of drugs in the human intestine. In order to solve this problem, we decided to use stem cell induction method to construct a simulated human intestinal environment, in which drug experiments can be conducted. In order to reduce the need for subsequent manual experiments to evaluate the antimicrobial effect, we designed an electronic measurement system to roughly estimate the distribution of colonies in each part of the Petri dish by the size of the colonies and the use of fluorescently labeled antimicrobial peptide images, so as to roughly assess the antimicrobial effect of the drug.
In order to evaluate the antimicrobial effect of the drug, we hope that the drug can attach to the gastrointestinal mucosa to fully carry out various biochemical reactions; It is hoped that the antimicrobial effect of the drug can be roughly evaluated by measuring the relationship between the concentration of Staphylococcus aureus and the change of the drug before and after administration with time
Build of Hardware System
Appearance
To implement calculating the distribution on various parts inside the petri dish by using colony
distribution image, an acquisition of image is required. For this, we designed a mini test
chambers which the size can contain a petri dish. Furthermore, a camera module, which can
fetch image data, connect to PC and can be controlled via microcontroller, is installed on the
top
of chamber.
This hardware design is inspired by the project described in this page:
(https://2022.igem.wiki/insa-lyon1/hardware)
The main structure is similar to the project inside the link. Nowadays, there is a team in iGEM designed a mini test chamber that fetches the colony distribution image by recording fluorescence information inside the petri dish. After fetching, the image data can be processed and analyzed by using PC.
Our electronic measuring system is a enhanced version of the project inside the link. We hope
that our enhanced hardware can fetch the image not only in the dark but also in the light.
Furthermore, we designed a sample algorithm to process colony distribution image by using
digital image processing (DIP) technology, as an attachment of this hardware design.
The following is the sketch of the mini test chamber:
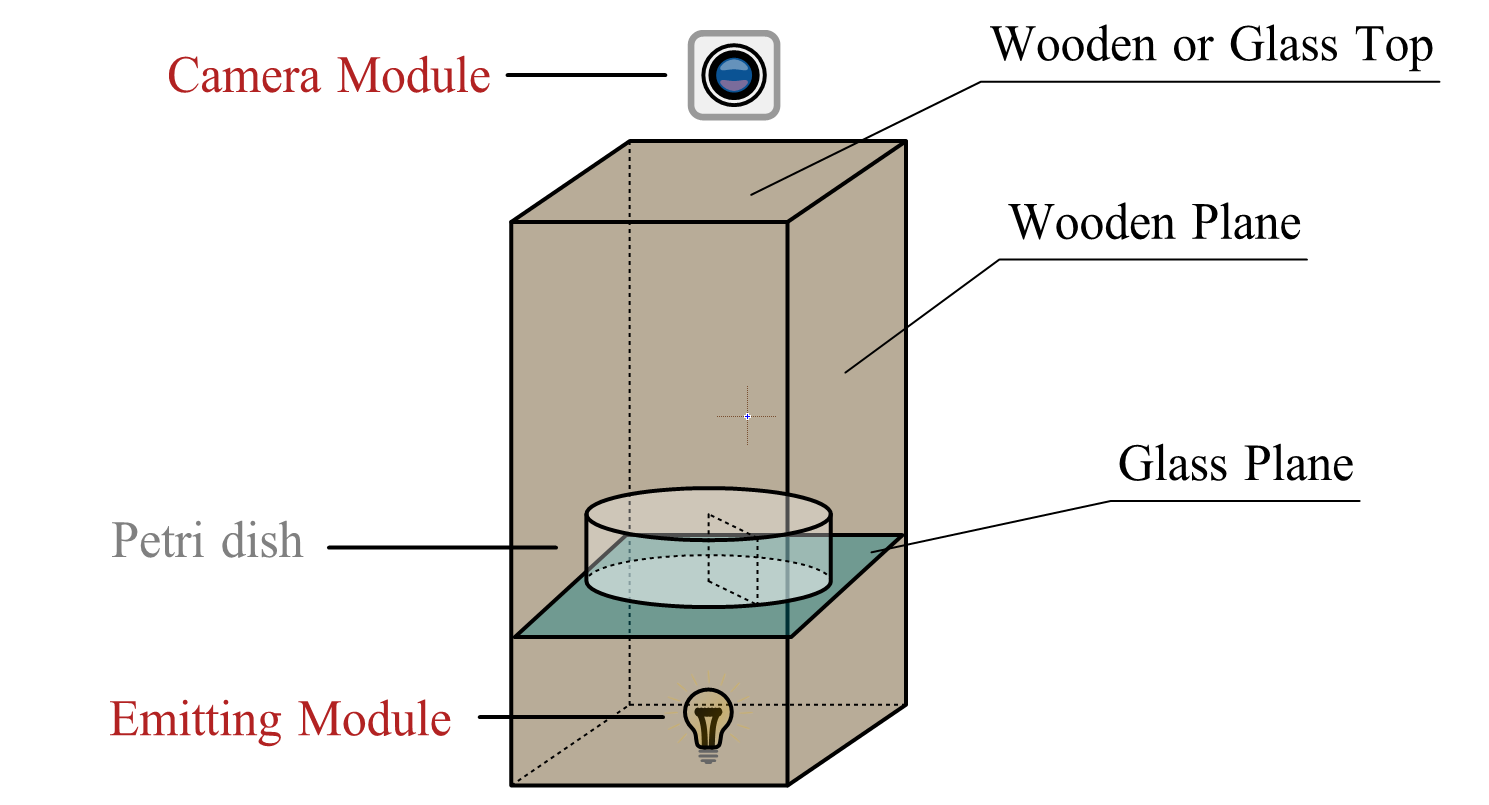
· Outside: Surrounded by wooden planes. The top is wooden or glass, and there is a joint to connect camera module.
· Wooden top: Isolate ambient light for measuring the distribution of colonies by using
fluorescence in the dark.
· Glass top: The light enters the test chamber from the top, for measurement of
colonied
that is colored or has a high contrast on the incubation medium.
· Inside: A glass plane is placed to place the petri dish.
· Camera Module: Fetching the distribution of colonies.
· Emitting Module: A light source controlled by a digital switch. Emits light of a
specific
wavelength to excite the fluorescence protein.
The following is the 3-D model of hardware appearance made by SketchUp. There is a placket in a side for facilitating the place of petri dish. The top model was not modelled.
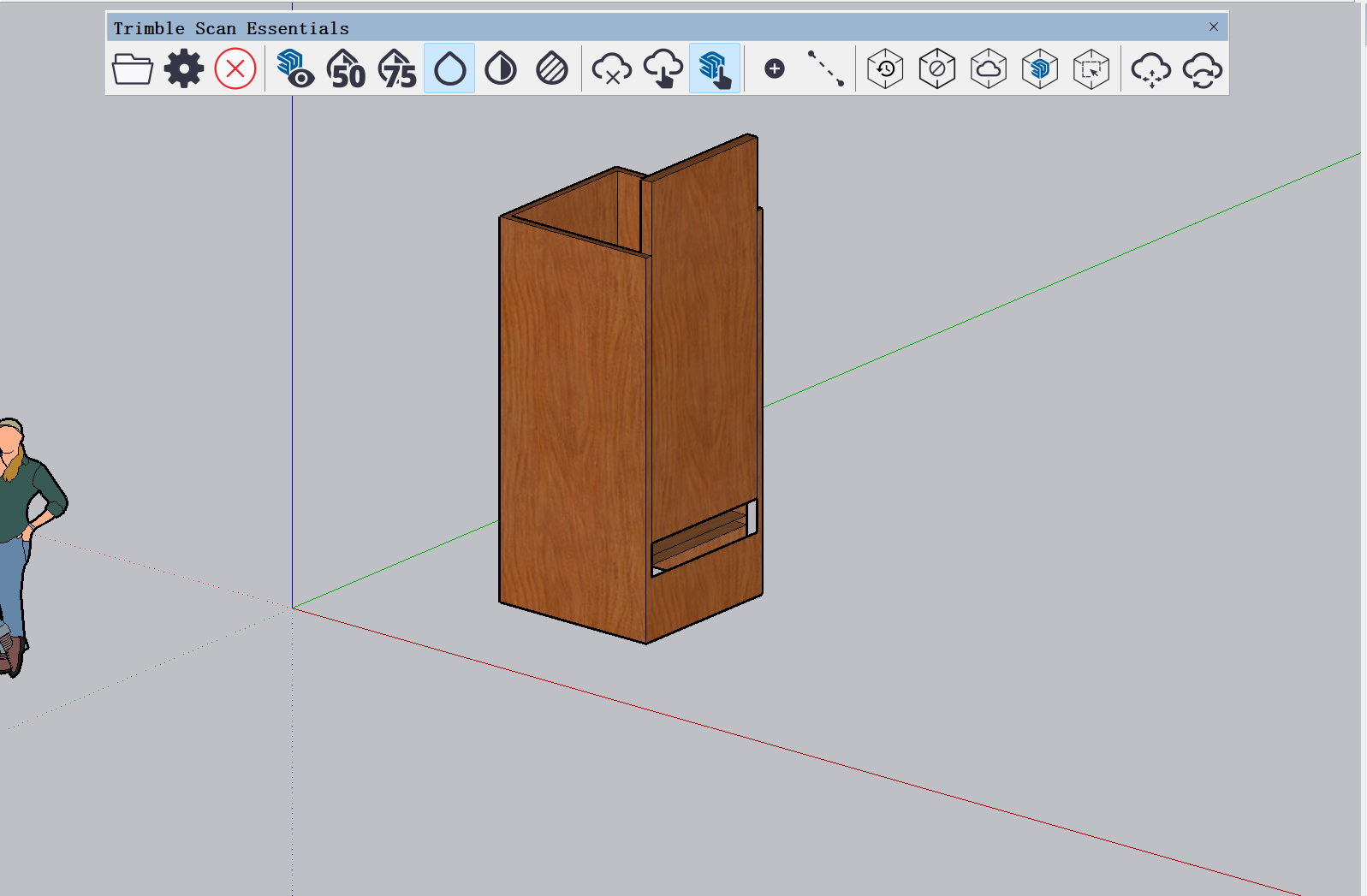
The following is the perspective structure of 3-D model. A glass plane is placed to place the petri dish.

Circuit Design
We designed a circuit to drive the camera and emitting module. The following is the block diagram of hardware circuit:
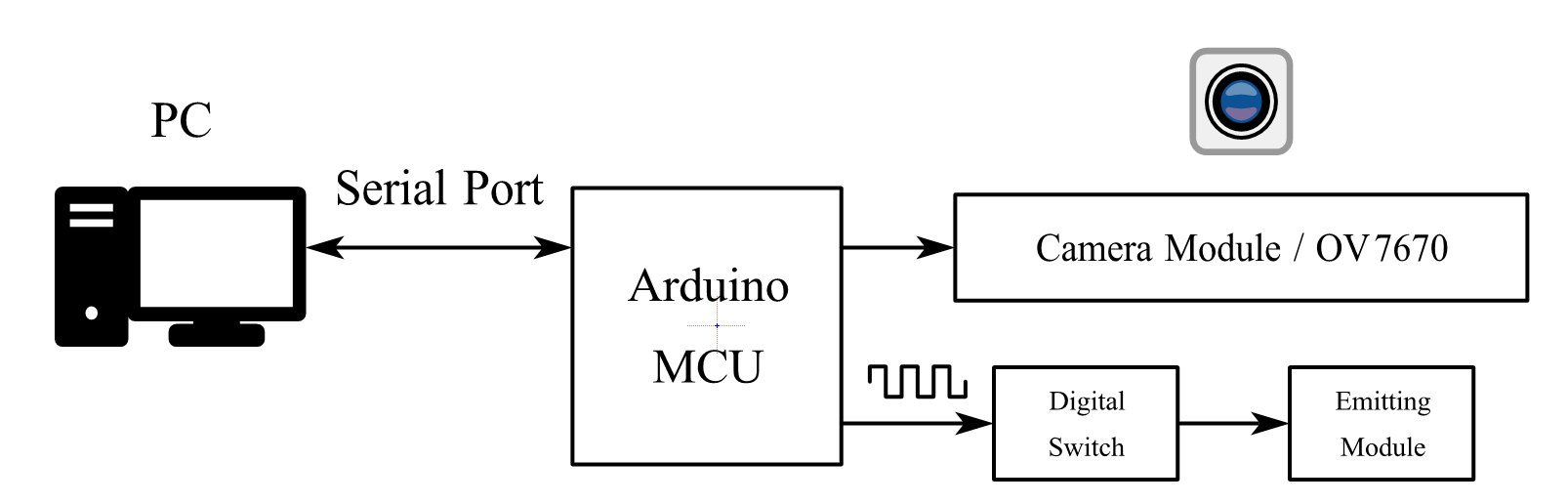
· Microcontroller Unit (MCU): The core of circuit. Arduino series MCU is selected, because
the
· programming of Arduino series MCU is simple, and there are a large number of
opensourced
library to drive various peripherals, and has a short developing period and high
efficiency.
· Camera Module: Using OV7670 Camera Module. It has a small size, simple structure, and
open-sourced driver code, which does not occupy the serial port.
· Emitting Module: The core of this module is a lamp that emits light of a specific
wavelength.
This module can be controlled by the digital pulse from MCU.
· PC: Processes the image information from MCU serial port.
The following is the schematic diagram of the circuit:
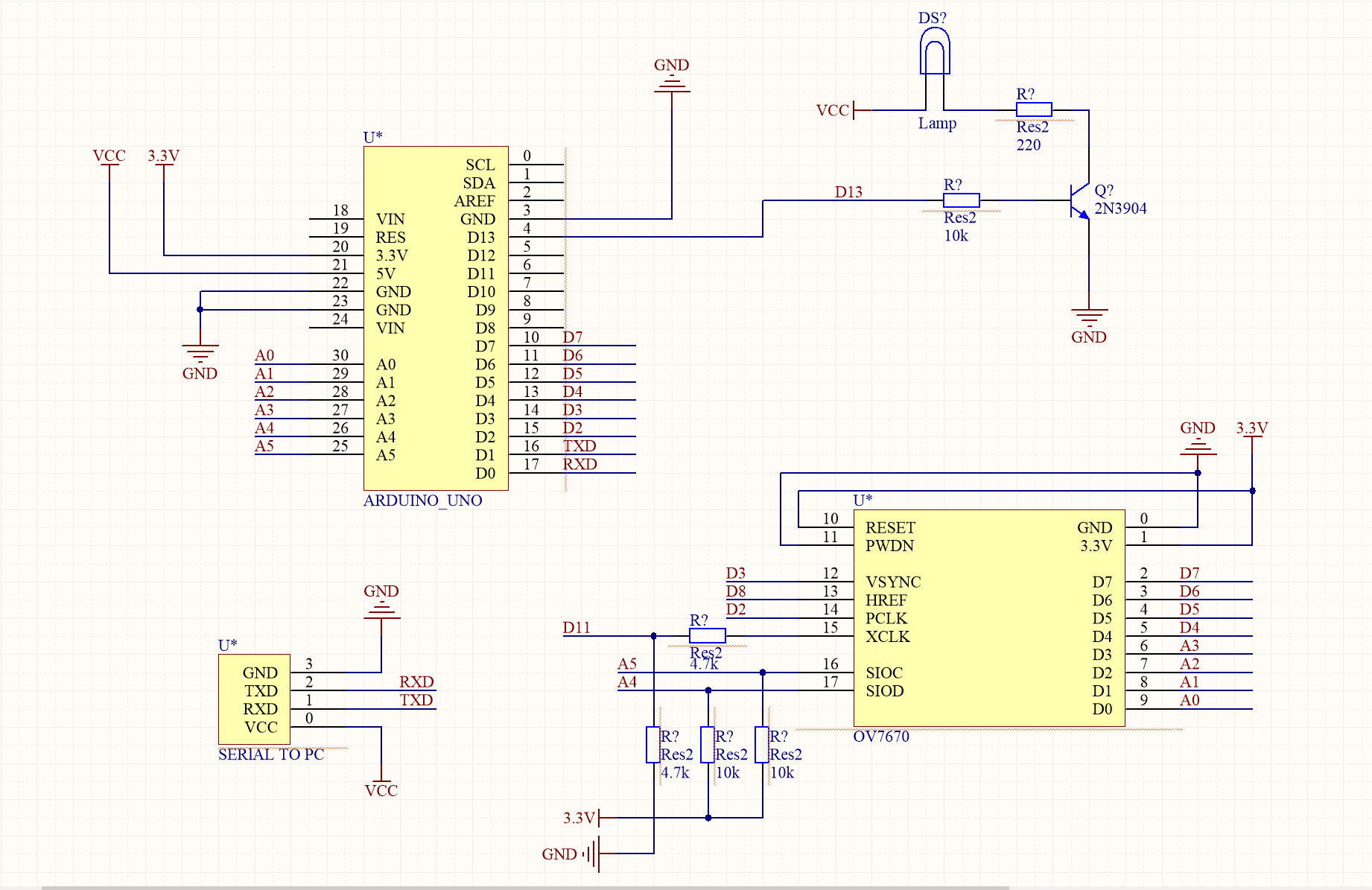
Software Introduction
After building the hardware system, the image from hardware system can be processed by PC now. We provided an algorithm based on DIP and breadth-first search (BFS). It calculates the ratio the area occupied by the colonies on the petri dish in a specific time approximately, based on the color of colonies or the excited fluorescence protein inside the bacteria itself. Based on this data, the survival of colonies can be tracked. Furthermore, the effect of the medicine can be evaluated approximately.
Image Processing
To suppress the influence of various errors, the image from MCU may be preprocessed. The following is a possible approach for processing and its implementation (in Python):
· Convert the image from the MCU (possibly in color) to 256-level grayscale image to reduce
the amount of computations;
· Save the grayscale image data in a file.
from PIL import Image from numpy import * from matplotlib.pyplot import * def imageProcessing(): # Read the image and convert it to an grayscale image img = Image.open("sample.png").convert("L") img_arr = array(img) # Save the grayscale image data savetxt("processed_img.txt", img_arr, fmt="%3d", delimiter=" ") # plot the grayscale image (optional) figure() imshow(img_arr, cmap="gray") show() return img_arr
In this example, our goal is to calculate fluorescence distribute area, and assuming that the image is acquired in the dark.
In an ideal case, place where has no fluorescence should be completely black, or the grayscale of there should be zero. However, during the analysis, the grayscale value where it looks like no fluorescence is a small value (about 0 ~ 10). To suppress the influence of these value, apply the Gamma Transform as the equation below:
$$g(x,y)=c * f(x, y) ^ {\gamma}$$Where
· \(g(x,y)\)、\(f(x,y)\) : The grayscale after and before the transform in location \((x, y)\).
The
grayscale is between 0 and 1.
· \(c\): An scale factor that can be customized.
· \(\gamma\): Gamma factor that can be customized too. Changing this factor may affect the
transformation result significantly. Especially,
· \(\gamma > 1\): Will suppress the low-value grayscale,
· \(\gamma < 1\): Will amplify the low-value grayscale.
def gammaTransform(img, c: float, gamma: float): for i in range(len(img)): for j in range(len(img[0])): # Convert the grayscale value to range between 0 and 1 img[i][j] = c * pow(img[i][j] / 255, gamma) * 255 return img
The following are the original colored image, original grayscale image and the image after Gamma Transform with \(c=1,\gamma=1.8\):
Due to the lack of related experiment, the image is from the links below for the
convenience to
describe the algorithm:
(https://2022.igem.wiki/insa-lyon1/hardware)
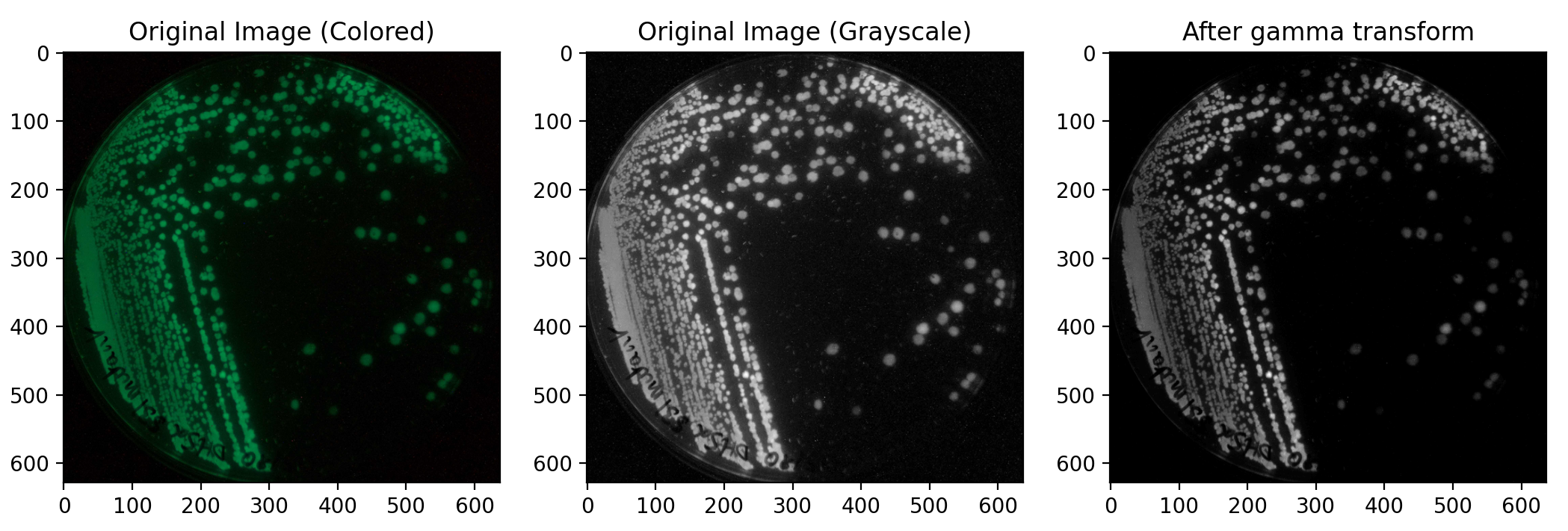
Algorithm Example: Estimate the Area of Fluorescence
After processing the image, the algorithm based on BFS can be applied to estimate the area of fluorescence:
· After processing, the following is how image may looks like (just a sketch):
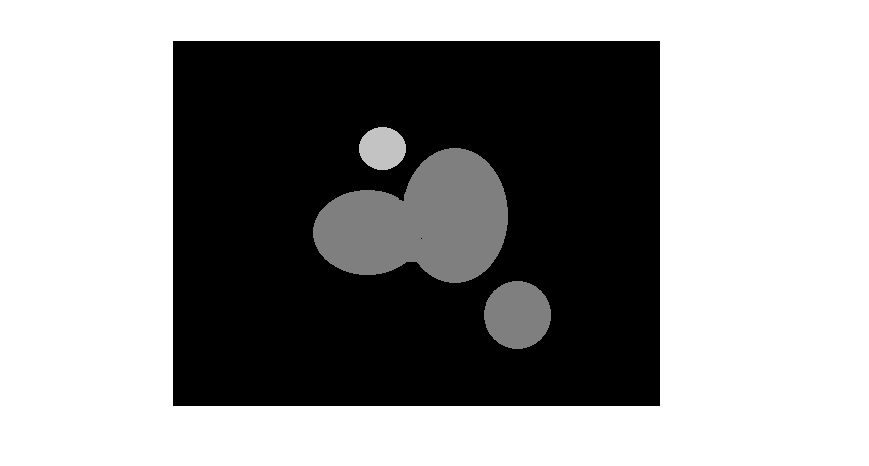
· To estimate the area of fluorescence in the petri dish, we can calculate the amount of light
pixel (for example, grayscale > 10), which corresponding to where the colonies locate.
· Enumerate all pixels. if the grayscale of a pixel is greater than 10, that means it may be
a
part of colony. Find the pixels near it and mark them as searched. After an enumeration, an
area can be calculated.
· Repeat the steps below until every pixels are enumerated.
The following is the implementation of this algorithm (in Python):
import numpy as np # An approach to find the covered area by using breadth-first search(BFS) algorithm def findArea(map, size_x:int, size_y:int, start_x:int, start_y:int): # directions for searching the neighbor of a specified point directions = [ Point(1, 0), Point(0, 1), Point(-1, 0), Point(0, -1) ] # whether the point has enqueued or not isEnqueued = np.zeros((size_x, size_y), dtype=bool) # build and initialize a queue for BFS algorithm points = Queue() points.enqueue(Point(start_x, start_y)) isEnqueued[start_x][start_y] = True # results of area calculation result = 0 while not points.isEmpty(): point = points.dequeue() result += 1 isEnqueued[point.getX()][point.getY()] = True # find appropriate parts of area and add them to query queue # and check whether the next point is inside the image for direction in directions: new_x = point.getX() + direction.getX() new_y = point.getY() + direction.getY() if (0 <= new_x and new_x < size_x) and (0 <= new_y and new_y < size_y): if not isEnqueued[new_x][new_y] and map[new_x][new_y] > 0: isEnqueued[new_x][new_y] = True points.enqueue(Point(new_x, new_y)) return result, isEnqueued def main(): # load the processed images data from the specified file. # the data will be a matrix, where each element is the gray scale of the # original image, and so on. map = np.loadtxt(" processed_img.txt" ,dtype=int) size_x = len(map) # the number of lines in the image size_y = len(map[0]) # the number of columns in the image # whether the point has enqueued or not isEnqueued = np.zeros((size_x, size_y), dtype=bool) result = 0 # find all possible areas inside the image for i in range(0, size_x): for j in range(0, size_y): if map[i][j] > 0 and (not isEnqueued[i][j]): tmp_result, isEnqueued = findArea(map, size_x, size_y, i, j) result = result + tmp_result print(result) if __name__ == ' __main__' : main()
Where Point is a class to store the location of pixels, and class Queue implements the queue data structure:
# A class which represents a point in the image class Point: def __init__(self): self.x = 0 self.y = 0 def __init__(self, x:int, y:int): self.x = x self.y = y # the add operation is overloaded for convenience of calculation def __add__(self, other): return Point(self.x + other.x, self.y + other.y) def getX(self): return self.x def getY(self): return self.y def toString(self): return " (" + str(self.x) + " , " + str(self.y) + " )"
# A simple implementation of the queue data structure class Queue: def __init__(self): self.items = [] def enqueue(self, item): self.items.append(item) def dequeue(self): return self.items.pop(0) def peek(self): return self.items[0] def isEmpty(self): return len(self.items) == 0
To estimate the total concentration of colonies, the algorithm can be improved as:
To estimate the total concentration of colonies, the algorithm can be improved as:
· Firstly calculate the cross-sectonal area \(S\), and acquire the image resolution
\(M\times
N\), thus the area of every pixel is \(S_0=\dfrac{S}{M\times N}\);
· Using the hardware to measure the (maximum) grayscale \(G\) by using the excited
fluorescence colonies, which concentrate given as \(c\) mol/L;
· Using DIP method to transform the grayscale in \([0, G]\) to \([0, 255]\) during the
follow-up
experiments;
· Assuming that the concentrate and the grayscale varies with a linear regularity, or
the
grayscale \(g(x, y)\) corresponds concentrate \(c\). Thus $$c(x,y)=\frac{G}{255}g(x,y)$$
· Finally, modify the area calculating program to estimate the total concentration of
colonies.
just change result += 1
to result += c[x][y]
inside the
while
in the function
findArea()
.
Conclusion
As an auxiliary biological experiment system, this hardware system can use computer and image processing methods to roughly count the distribution of colonies. In order to count the colonization efficiency of drugs in the intestine, the excitation module of this module can be used to excite the fluorescent protein inside the antimicrobial peptide, and then the colony distribution can be estimated by computer. To evaluate the killing effect of antimicrobial peptides against Staphylococcus aureus, natural light can be used instead of a dark environment, as Staphylococcus aureus is inherently colored, and the same method can be used to estimate colony distribution.